Magic Tables are special tables in SQL Server that are used in triggers to access the data being inserted, updated, or deleted. They are called INSERTED and DELETED tables. In this blog post, we'll explore what Magic Tables are, how they work, and how you can use them in ASP.NET applications.
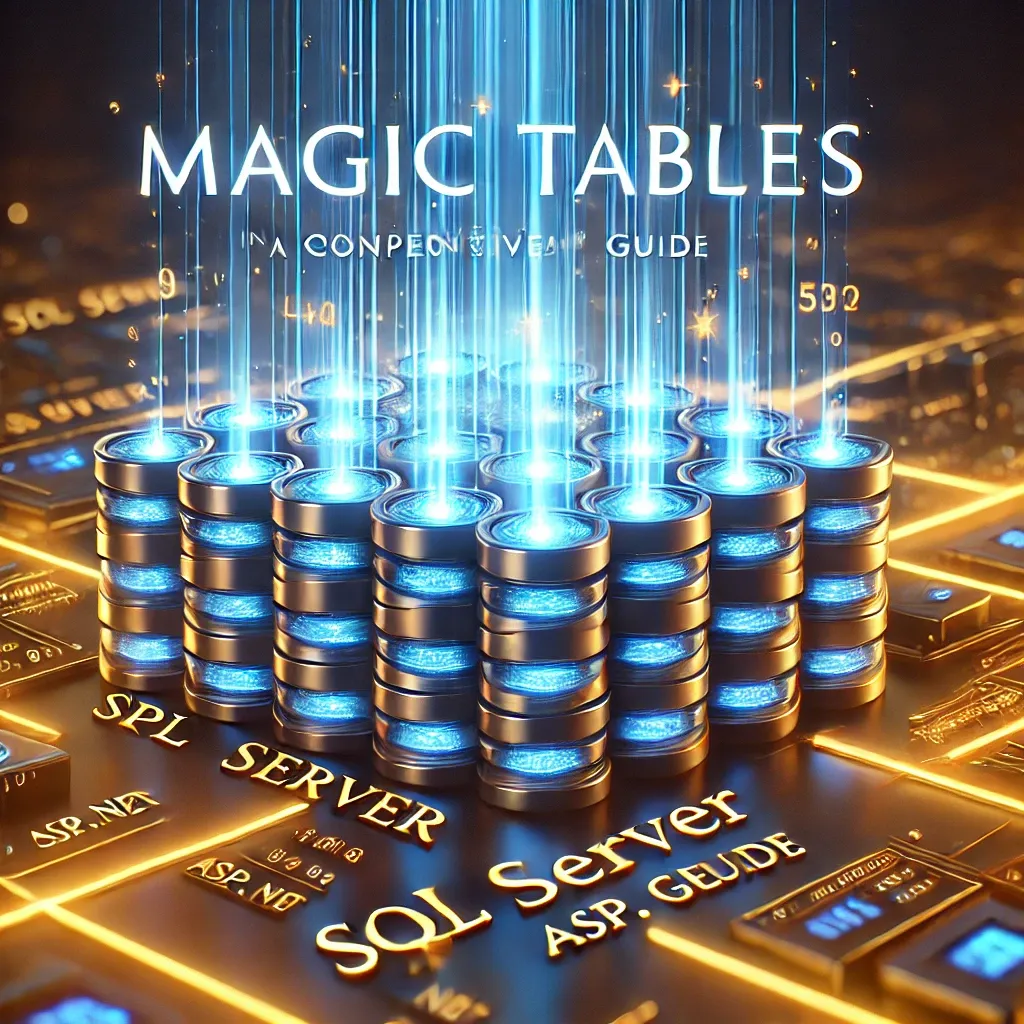
What are Magic Tables?
Magic Tables are temporary tables that SQL Server automatically creates during the execution of a trigger. They hold the data that is being affected by the trigger. There are two types of Magic Tables:
- INSERTED: Contains the new data for
INSERT
andUPDATE
operations. - DELETED: Contains the old data for
DELETE
andUPDATE
operations.
How Magic Tables Work
When a trigger is fired, SQL Server creates the INSERTED
and DELETED
tables to store the affected data. These tables have the same structure as the table on which the trigger is defined.
Example: Using Magic Tables in a Trigger
Let's create a trigger that logs changes to a table into an audit table using Magic Tables.
Step 1: Create the Main Table
CREATE TABLE Employees (
ID INT PRIMARY KEY,
Name NVARCHAR(50),
Age INT,
Department NVARCHAR(50)
);
Step 2: Create the Audit Table
CREATE TABLE EmployeeAudit (
AuditID INT IDENTITY PRIMARY KEY,
EmployeeID INT,
Action NVARCHAR(10),
ActionDate DATETIME
);
Step 3: Create the Trigger
CREATE TRIGGER trg_EmployeeAudit
ON Employees
FOR INSERT, UPDATE, DELETE
AS
BEGIN
-- Log INSERT operations
IF EXISTS (SELECT * FROM INSERTED) AND NOT EXISTS (SELECT * FROM DELETED)
BEGIN
INSERT INTO EmployeeAudit (EmployeeID, Action, ActionDate)
SELECT ID, 'INSERT', GETDATE() FROM INSERTED;
END
-- Log DELETE operations
IF EXISTS (SELECT * FROM DELETED) AND NOT EXISTS (SELECT * FROM INSERTED)
BEGIN
INSERT INTO EmployeeAudit (EmployeeID, Action, ActionDate)
SELECT ID, 'DELETE', GETDATE() FROM DELETED;
END
-- Log UPDATE operations
IF EXISTS (SELECT * FROM INSERTED) AND EXISTS (SELECT * FROM DELETED)
BEGIN
INSERT INTO EmployeeAudit (EmployeeID, Action, ActionDate)
SELECT ID, 'UPDATE', GETDATE() FROM INSERTED;
END
END;
Using Magic Tables in ASP.NET
In an ASP.NET application, you can use Magic Tables indirectly by executing SQL queries that involve triggers. Below is an example of how to perform CRUD operations in ASP.NET and log changes using the trigger we created earlier.
Step 1: Perform CRUD Operations
Use ADO.NET or Entity Framework to perform CRUD operations on the Employees
table.
Example: Inserting a Record
using System;
using System.Data.SqlClient;
public partial class EmployeeManagement : System.Web.UI.Page
{
protected void btnInsert_Click(object sender, EventArgs e)
{
string connectionString = "Your_Connection_String";
string query = "INSERT INTO Employees (ID, Name, Age, Department) VALUES (@ID, @Name, @Age, @Department)";
using (SqlConnection con = new SqlConnection(connectionString))
{
using (SqlCommand cmd = new SqlCommand(query, con))
{
cmd.Parameters.AddWithValue("@ID", 1);
cmd.Parameters.AddWithValue("@Name", "John Doe");
cmd.Parameters.AddWithValue("@Age", 25);
cmd.Parameters.AddWithValue("@Department", "IT");
con.Open();
cmd.ExecuteNonQuery();
}
}
}
}
Step 2: View Audit Logs
You can retrieve the audit logs from the EmployeeAudit
table and display them in a GridView.
Example: Displaying Audit Logs
using System;
using System.Data;
using System.Data.SqlClient;
using System.Web.UI.WebControls;
public partial class AuditLogs : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
string connectionString = "Your_Connection_String";
string query = "SELECT * FROM EmployeeAudit";
using (SqlConnection con = new SqlConnection(connectionString))
{
using (SqlCommand cmd = new SqlCommand(query, con))
{
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataTable dt = new DataTable();
da.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
}
}
Conclusion
Magic Tables are a powerful feature in SQL Server that allow you to access the data being affected by INSERT
, UPDATE
, and DELETE
operations in triggers. By using Magic Tables, you can implement auditing, logging, and other advanced functionalities in your ASP.NET applications. Experiment with the examples provided and explore more advanced use cases to enhance your skills.
Note: Replace Your_Connection_String
with actual values.