Mastering GridView in C# and .NET
GridView is one of the most powerful and widely used controls in ASP.NET for displaying and managing tabular data. Whether you're building a simple data table or a complex data-driven web application, GridView provides a flexible and feature-rich solution. In this blog post, we'll dive deep into GridView, exploring its features, usage, and examples.
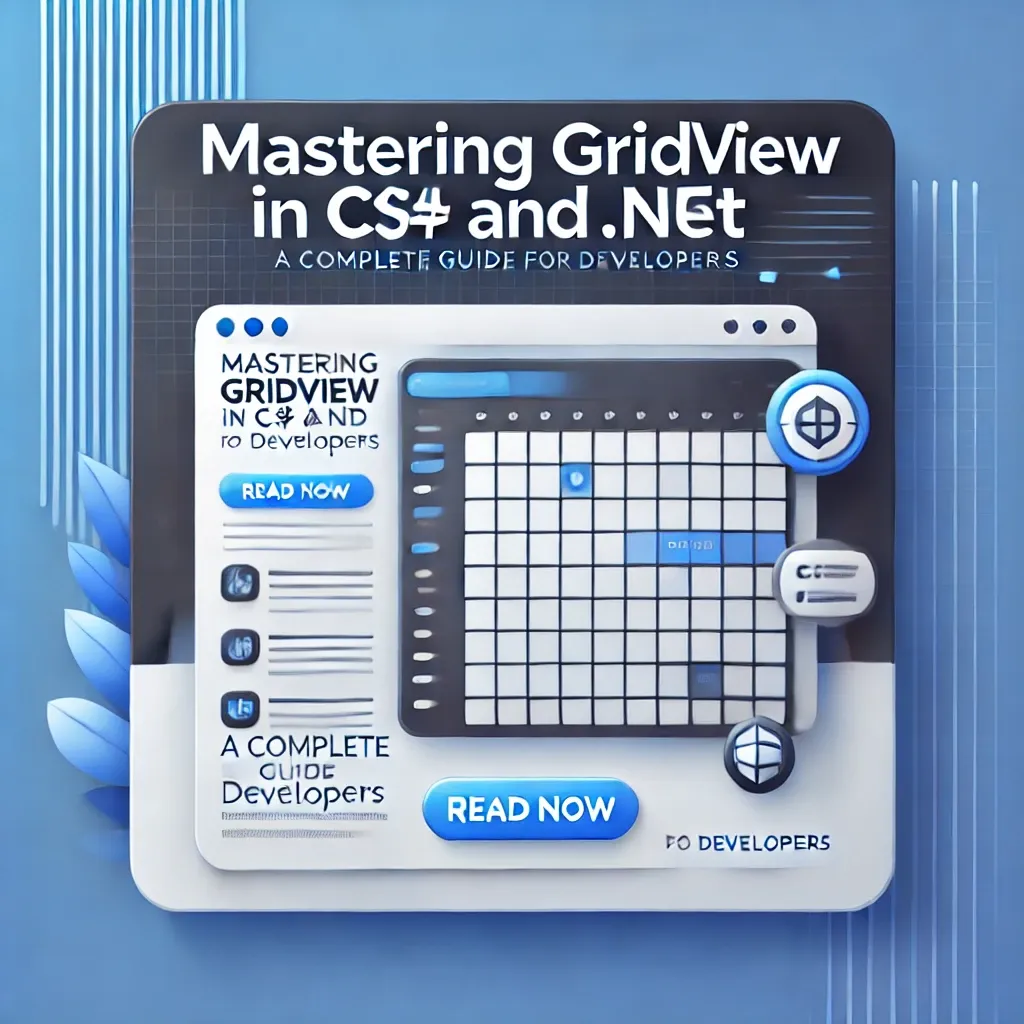
What is GridView?
GridView is a server-side control in ASP.NET that allows you to display data in a tabular format. It supports features like sorting, paging, editing, and deleting data. GridView is highly customizable and can be bound to various data sources, such as databases, XML files, or collections.
Key Features of GridView
- Data Binding: Bind GridView to data sources like SQL Server, XML, or collections.
- Sorting: Allow users to sort data by clicking on column headers.
- Paging: Display data in pages to improve performance and usability.
- Editing and Deleting: Enable inline editing and deletion of records.
- Customization: Customize the appearance using templates and styles.
- Events: Handle events like RowDataBound, RowCommand, and PageIndexChanging.
Basic Example of GridView
Let's start with a simple example of binding a GridView to a data source.
Step 1: Create a Data Table
First, create a sample data table in your C# code-behind file.
using System;
using System.Data;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
DataTable dt = new DataTable();
dt.Columns.Add("ID", typeof(int));
dt.Columns.Add("Name", typeof(string));
dt.Columns.Add("Age", typeof(int));
dt.Rows.Add(1, "John Doe", 25);
dt.Rows.Add(2, "Jane Smith", 30);
dt.Rows.Add(3, "Sam Brown", 22);
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
Step 2: Add GridView to ASP.NET Page
Add the GridView control to your ASP.NET page.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true">
</asp:GridView>
Advanced Features of GridView
Now that you've seen a basic example, let's explore some advanced features.
1. Custom Columns
You can define custom columns in GridView for better control over the layout and data display.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false">
<Columns>
<asp:BoundField DataField="ID" HeaderText="ID" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Age" HeaderText="Age" />
</Columns>
</asp:GridView>
2. Sorting
Enable sorting by setting the AllowSorting
property to true
and handling the Sorting
event.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true" AllowSorting="true" OnSorting="GridView1_Sorting">
</asp:GridView>
In the code-behind:
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
// Implement sorting logic here
}
3. Paging
Enable paging by setting the AllowPaging
property to true
and handling the PageIndexChanging
event.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true" AllowPaging="true" PageSize="5" OnPageIndexChanging="GridView1_PageIndexChanging">
</asp:GridView>
In the code-behind:
protected void GridView1_PageIndexChanging(object sender, GridViewPageEventArgs e)
{
GridView1.PageIndex = e.NewPageIndex;
BindGridView();
}
4. Editing and Deleting
Enable editing and deleting by adding CommandField
columns and handling the RowEditing
, RowUpdating
, and RowDeleting
events.
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" OnRowEditing="GridView1_RowEditing" OnRowUpdating="GridView1_RowUpdating" OnRowDeleting="GridView1_RowDeleting">
<Columns>
<asp:BoundField DataField="ID" HeaderText="ID" ReadOnly="true" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Age" HeaderText="Age" />
<asp:CommandField ShowEditButton="true" ShowDeleteButton="true" />
</Columns>
</asp:GridView>
In the code-behind:
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
BindGridView();
}
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
// Implement update logic here
GridView1.EditIndex = -1;
BindGridView();
}
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
// Implement delete logic here
BindGridView();
}
Data Binding in GridView
Data binding is one of the most fundamental features of the GridView control in ASP.NET. It allows you to connect the GridView to various data sources, such as databases, XML files, or in-memory collections, and display the data in a tabular format. In this blog post, we'll explore how to bind GridView to different data sources with detailed examples.
What is Data Binding?
Data binding is the process of connecting a control (like GridView) to a data source and automatically displaying the data. GridView supports binding to various data sources, including:
- Databases (e.g., SQL Server, MySQL)
- XML Files
- Collections (e.g., List, DataTable)
1. Binding GridView to a SQL Server Database
Binding GridView to a SQL Server database is a common use case. Below is a step-by-step example.
Step 1: Create a Database Table
First, create a sample table in your SQL Server database. For example:
CREATE TABLE Employees (
ID INT PRIMARY KEY,
Name NVARCHAR(50),
Age INT,
Department NVARCHAR(50)
);
Step 2: Insert Sample Data
Insert some sample data into the table:
INSERT INTO Employees (ID, Name, Age, Department)
VALUES
(1, 'John Doe', 25, 'IT'),
(2, 'Jane Smith', 30, 'HR'),
(3, 'Sam Brown', 22, 'Finance');
Step 3: Bind GridView to the Database
Now, let's bind the GridView to the database in your ASP.NET application.
using System;
using System.Data;
using System.Data.SqlClient;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
string connectionString = "Your_Connection_String";
string query = "SELECT * FROM Employees";
using (SqlConnection con = new SqlConnection(connectionString))
{
SqlDataAdapter da = new SqlDataAdapter(query, con);
DataTable dt = new DataTable();
da.Fill(dt);
GridView1.DataSource = dt;
GridView1.DataBind();
}
}
}
Step 4: Add GridView to ASP.NET Page
Add the GridView control to your ASP.NET page:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true">
</asp:GridView>
2. Binding GridView to an XML File
GridView can also be bound to an XML file. Below is an example.
Step 1: Create an XML File
Create an XML file named Employees.xml
:
<Employees>
<Employee>
<ID>1</ID>
<Name>John Doe</Name>
<Age>25</Age>
<Department>IT</Department>
</Employee>
<Employee>
<ID>2</ID>
<Name>Jane Smith</Name>
<Age>30</Age>
<Department>HR</Department>
</Employee>
<Employee>
<ID>3</ID>
<Name>Sam Brown</Name>
<Age>22</Age>
<Department>Finance</Department>
</Employee>
</Employees>
Step 2: Bind GridView to the XML File
Bind the GridView to the XML file in your code-behind:
using System;
using System.Data;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
DataSet ds = new DataSet();
ds.ReadXml(Server.MapPath("~/Employees.xml"));
GridView1.DataSource = ds.Tables[0];
GridView1.DataBind();
}
}
3. Binding GridView to a Collection
You can also bind GridView to an in-memory collection, such as a List
or DataTable
.
Example: Binding to a List
Create a list of objects and bind it to the GridView:
using System;
using System.Collections.Generic;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
List<Employee> employees = new List<Employee>
{
new Employee { ID = 1, Name = "John Doe", Age = 25, Department = "IT" },
new Employee { ID = 2, Name = "Jane Smith", Age = 30, Department = "HR" },
new Employee { ID = 3, Name = "Sam Brown", Age = 22, Department = "Finance" }
};
GridView1.DataSource = employees;
GridView1.DataBind();
}
}
public class Employee
{
public int ID { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public string Department { get; set; }
}
Data binding is a powerful feature of GridView that allows you to display data from various sources like SQL Server, XML files, and collections. By mastering data binding, you can create dynamic and data-driven web applications with ease. Experiment with the examples provided and explore more advanced features to enhance your skills.
Sorting in GridView
Sorting is a crucial feature in any data-driven application, allowing users to organize and view data in a meaningful way. In ASP.NET, the GridView control provides built-in support for sorting, making it easy to implement this functionality. In this blog post, we'll explore how to enable sorting in GridView, handle sorting events, and customize sorting behavior with detailed examples.
What is Sorting in GridView?
Sorting in GridView allows users to sort data by clicking on column headers. When a column header is clicked, the data is rearranged in ascending or descending order based on the selected column. GridView supports both automatic and custom sorting.
1. Enabling Sorting in GridView
To enable sorting in GridView, you need to set the AllowSorting
property to true
and handle the Sorting
event.
Step 1: Set AllowSorting Property
Add the GridView control to your ASP.NET page and set the AllowSorting
property:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true" AllowSorting="true" OnSorting="GridView1_Sorting">
</asp:GridView>
Step 2: Handle the Sorting Event
In the code-behind, handle the Sorting
event to implement sorting logic:
using System;
using System.Data;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
DataTable dt = GetData(); // Fetch data from your data source
GridView1.DataSource = dt;
GridView1.DataBind();
}
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
DataTable dt = GetData(); // Fetch data from your data source
dt.DefaultView.Sort = e.SortExpression + " " + GetSortDirection(e.SortExpression);
GridView1.DataSource = dt;
GridView1.DataBind();
}
private string GetSortDirection(string column)
{
string sortDirection = "ASC";
if (ViewState["SortExpression"] != null && ViewState["SortExpression"].ToString() == column)
{
sortDirection = (ViewState["SortDirection"].ToString() == "ASC") ? "DESC" : "ASC";
}
ViewState["SortExpression"] = column;
ViewState["SortDirection"] = sortDirection;
return sortDirection;
}
private DataTable GetData()
{
// Sample data for demonstration
DataTable dt = new DataTable();
dt.Columns.Add("ID", typeof(int));
dt.Columns.Add("Name", typeof(string));
dt.Columns.Add("Age", typeof(int));
dt.Rows.Add(1, "John Doe", 25);
dt.Rows.Add(2, "Jane Smith", 30);
dt.Rows.Add(3, "Sam Brown", 22);
return dt;
}
}
2. Customizing Sorting Behavior
By default, GridView sorts data in ascending order when a column header is clicked for the first time and toggles between ascending and descending order on subsequent clicks. You can customize this behavior to suit your needs.
Example: Custom Sort Direction
In the GetSortDirection
method, you can customize the sort direction logic. For example, you can always start with descending order:
private string GetSortDirection(string column)
{
string sortDirection = "DESC"; // Always start with descending order
if (ViewState["SortExpression"] != null && ViewState["SortExpression"].ToString() == column)
{
sortDirection = (ViewState["SortDirection"].ToString() == "DESC") ? "ASC" : "DESC";
}
ViewState["SortExpression"] = column;
ViewState["SortDirection"] = sortDirection;
return sortDirection;
}
3. Sorting with Custom Columns
If you're using custom columns in GridView (e.g., TemplateField
or BoundField
), you need to specify the SortExpression
for each column.
Example: Sorting with BoundField
Add BoundField
columns and set the SortExpression
property:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" AllowSorting="true" OnSorting="GridView1_Sorting">
<Columns>
<asp:BoundField DataField="ID" HeaderText="ID" SortExpression="ID" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
<asp:BoundField DataField="Age" HeaderText="Age" SortExpression="Age" />
</Columns>
</asp:GridView>
4. Sorting with Complex Data
If your data source contains complex objects (e.g., a list of custom objects), you can implement custom sorting logic in the Sorting
event.
Example: Sorting a List of Custom Objects
Create a list of custom objects and sort it based on the selected column:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
List<Employee> employees = new List<Employee>
{
new Employee { ID = 1, Name = "John Doe", Age = 25 },
new Employee { ID = 2, Name = "Jane Smith", Age = 30 },
new Employee { ID = 3, Name = "Sam Brown", Age = 22 }
};
GridView1.DataSource = employees;
GridView1.DataBind();
}
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
List<Employee> employees = GetEmployees(); // Fetch data
var sortedEmployees = employees.OrderBy(e.SortExpression).ToList(); // Sort data
if (ViewState["SortDirection"] != null && ViewState["SortDirection"].ToString() == "DESC")
{
sortedEmployees = employees.OrderByDescending(e.SortExpression).ToList();
}
GridView1.DataSource = sortedEmployees;
GridView1.DataBind();
}
private List<Employee> GetEmployees()
{
// Sample data for demonstration
return new List<Employee>
{
new Employee { ID = 1, Name = "John Doe", Age = 25 },
new Employee { ID = 2, Name = "Jane Smith", Age = 30 },
new Employee { ID = 3, Name = "Sam Brown", Age = 22 }
};
}
}
public class Employee
{
public int ID { get; set; }
public string Name { get; set; }
public int Age { get; set; }
}
Paging in GridView
Paging is a critical feature in data-driven applications, especially when dealing with large datasets. It allows you to display data in smaller, more manageable chunks (pages) rather than loading all records at once. In ASP.NET, the GridView control provides built-in support for paging, making it easy to implement this functionality. In this blog post, we'll explore how to enable paging in GridView, customize page size, handle paging events, and implement efficient data fetching with detailed examples.
What is Paging in GridView?
Paging in GridView divides a large dataset into multiple pages, allowing users to navigate through the data using page numbers or navigation buttons. This improves performance and usability by reducing the amount of data loaded at once.
1. Enabling Paging in GridView
To enable paging in GridView, you need to set the AllowPaging
property to true
and handle the PageIndexChanging
event.
Step 1: Set AllowPaging Property
Add the GridView control to your ASP.NET page and set the AllowPaging
property:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true" AllowPaging="true" PageSize="5" OnPageIndexChanging="GridView1_PageIndexChanging">
</asp:GridView>
Step 2: Handle the PageIndexChanging Event
In the code-behind, handle the PageIndexChanging
event to update the current page index and rebind the data:
using System;
using System.Data;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
DataTable dt = GetData(); // Fetch data from your data source
GridView1.DataSource = dt;
GridView1.DataBind();
}
protected void GridView1_PageIndexChanging(object sender, GridViewPageEventArgs e)
{
GridView1.PageIndex = e.NewPageIndex;
BindGridView();
}
private DataTable GetData()
{
// Sample data for demonstration
DataTable dt = new DataTable();
dt.Columns.Add("ID", typeof(int));
dt.Columns.Add("Name", typeof(string));
dt.Columns.Add("Age", typeof(int));
for (int i = 1; i <= 50; i++)
{
dt.Rows.Add(i, "User " + i, 20 + i);
}
return dt;
}
}
2. Customizing Page Size
You can customize the number of records displayed per page by setting the PageSize
property. For example, to display 10 records per page:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true" AllowPaging="true" PageSize="10" OnPageIndexChanging="GridView1_PageIndexChanging">
</asp:GridView>
3. Custom Pager Settings
GridView allows you to customize the pager settings, such as the position, mode, and text of the pager.
Example: Custom Pager Position and Mode
Set the PagerSettings
property to customize the pager:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true" AllowPaging="true" PageSize="5" OnPageIndexChanging="GridView1_PageIndexChanging">
<PagerSettings Position="TopAndBottom" Mode="NumericFirstLast" FirstPageText="First" LastPageText="Last" />
</asp:GridView>
4. Efficient Data Fetching for Large Datasets
When working with large datasets, fetching all records at once can be inefficient. Instead, you can fetch only the records for the current page using server-side pagination.
Example: Server-Side Pagination
Modify the GetData
method to fetch only the records for the current page:
private DataTable GetData()
{
int pageSize = GridView1.PageSize;
int pageIndex = GridView1.PageIndex;
int startIndex = pageIndex * pageSize + 1;
int endIndex = startIndex + pageSize - 1;
// Fetch only the records for the current page
DataTable dt = new DataTable();
dt.Columns.Add("ID", typeof(int));
dt.Columns.Add("Name", typeof(string));
dt.Columns.Add("Age", typeof(int));
for (int i = startIndex; i <= endIndex; i++)
{
dt.Rows.Add(i, "User " + i, 20 + i);
}
return dt;
}
5. Handling Paging Events
You can handle additional paging events, such as PageIndexChanged
, to perform custom logic when the page changes.
Example: Handling PageIndexChanged Event
Add the OnPageIndexChanged
event to the GridView:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="true" AllowPaging="true" PageSize="5" OnPageIndexChanging="GridView1_PageIndexChanging" OnPageIndexChanged="GridView1_PageIndexChanged">
</asp:GridView>
In the code-behind:
protected void GridView1_PageIndexChanged(object sender, EventArgs e)
{
// Custom logic after the page index has changed
// For example, display a message
Response.Write("Page changed to: " + (GridView1.PageIndex + 1));
}
Editing and Deleting in GridView
Editing and deleting records are essential features in any data-driven application. In ASP.NET, the GridView control provides built-in support for inline editing and deleting, making it easy to implement these functionalities. In this blog post, we'll explore how to enable editing and deleting in GridView, handle editing and deleting events, and customize the editing interface with detailed examples.
What are Editing and Deleting in GridView?
Editing and deleting in GridView allow users to modify or remove records directly from the GridView interface. When a user clicks the "Edit" button, the corresponding row switches to edit mode, displaying text boxes or other input controls. When the user clicks the "Delete" button, the corresponding record is removed from the data source.
1. Enabling Editing and Deleting in GridView
To enable editing and deleting in GridView, you need to add CommandField
columns and handle the RowEditing
, RowUpdating
, and RowDeleting
events.
Step 1: Add CommandField Columns
Add CommandField
columns to the GridView to enable editing and deleting:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" OnRowEditing="GridView1_RowEditing" OnRowUpdating="GridView1_RowUpdating" OnRowDeleting="GridView1_RowDeleting">
<Columns>
<asp:BoundField DataField="ID" HeaderText="ID" ReadOnly="true" />
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Age" HeaderText="Age" />
<asp:CommandField ShowEditButton="true" ShowDeleteButton="true" />
</Columns>
</asp:GridView>
Step 2: Handle Editing and Deleting Events
In the code-behind, handle the RowEditing
, RowUpdating
, and RowDeleting
events:
using System;
using System.Data;
using System.Web.UI.WebControls;
public partial class GridViewExample : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGridView();
}
}
private void BindGridView()
{
DataTable dt = GetData(); // Fetch data from your data source
GridView1.DataSource = dt;
GridView1.DataBind();
}
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex;
BindGridView();
}
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
// Get the updated values
string id = GridView1.DataKeys[e.RowIndex].Value.ToString();
string name = ((TextBox)GridView1.Rows[e.RowIndex].Cells[1].Controls[0]).Text;
string age = ((TextBox)GridView1.Rows[e.RowIndex].Cells[2].Controls[0]).Text;
// Update the data source (e.g., database)
UpdateData(id, name, age);
// Exit edit mode
GridView1.EditIndex = -1;
BindGridView();
}
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
// Get the ID of the record to delete
string id = GridView1.DataKeys[e.RowIndex].Value.ToString();
// Delete the record from the data source (e.g., database)
DeleteData(id);
// Rebind the GridView
BindGridView();
}
private DataTable GetData()
{
// Sample data for demonstration
DataTable dt = new DataTable();
dt.Columns.Add("ID", typeof(int));
dt.Columns.Add("Name", typeof(string));
dt.Columns.Add("Age", typeof(int));
dt.Rows.Add(1, "John Doe", 25);
dt.Rows.Add(2, "Jane Smith", 30);
dt.Rows.Add(3, "Sam Brown", 22);
return dt;
}
private void UpdateData(string id, string name, string age)
{
// Implement update logic (e.g., update database)
}
private void DeleteData(string id)
{
// Implement delete logic (e.g., delete from database)
}
}
2. Customizing the Editing Interface
You can customize the editing interface by using TemplateField
instead of BoundField
. This allows you to use different controls (e.g., dropdowns, checkboxes) for editing.
Example: Using TemplateField for Custom Controls
Replace BoundField
with TemplateField
to customize the editing interface:
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false" OnRowEditing="GridView1_RowEditing" OnRowUpdating="GridView1_RowUpdating" OnRowDeleting="GridView1_RowDeleting">
<Columns>
<asp:TemplateField HeaderText="ID">
<ItemTemplate>
<asp:Label ID="lblID" runat="server" Text='<%# Eval("ID") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Name">
<ItemTemplate>
<asp:Label ID="lblName" runat="server" Text='<%# Eval("Name") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox ID="txtName" runat="server" Text='<%# Bind("Name") %>'></asp:TextBox>
</EditItemTemplate>
</asp:TemplateField>
<asp:TemplateField HeaderText="Age">
<ItemTemplate>
<asp:Label ID="lblAge" runat="server" Text='<%# Eval("Age") %>'></asp:Label>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox ID="txtAge" runat="server" Text='<%# Bind("Age") %>'></asp:TextBox>
</EditItemTemplate>
</asp:TemplateField>
<asp:CommandField ShowEditButton="true" ShowDeleteButton="true" />
</Columns>
</asp:GridView>
3. Handling Validation During Editing
You can add validation controls (e.g., RequiredFieldValidator
) to ensure that users enter valid data during editing.
Example: Adding Validation Controls
Add validation controls to the EditItemTemplate
:
<EditItemTemplate>
<asp:TextBox ID="txtName" runat="server" Text='<%# Bind("Name") %>'></asp:TextBox>
<asp:RequiredFieldValidator ID="rfvName" runat="server" ControlToValidate="txtName" ErrorMessage="Name is required" Display="Dynamic"></asp:RequiredFieldValidator>
</EditItemTemplate>
4. Confirming Deletion
You can add a confirmation dialog to prevent accidental deletions.
Example: Adding a Confirmation Dialog
Use JavaScript to add a confirmation dialog:
<asp:CommandField ShowDeleteButton="true" />
<asp:TemplateField>
<ItemTemplate>
<asp:LinkButton ID="lnkDelete" runat="server" CommandName="Delete" OnClientClick="return confirm('Are you sure you want to delete this record?');" Text="Delete"></asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
Conclusion
Paging is a powerful feature of GridView that enhances the performance and usability of your data-driven applications. By enabling and customizing paging, you can provide users with a seamless experience for navigating through large datasets. Experiment with the examples provided and explore more advanced paging techniques to take your skills to the next level.
Note: This blog post assumes basic familiarity with C#, .NET, and ASP.NET. If you're new to these technologies, consider learning the basics before diving into GridView.